Slot Machine in Python. GitHub Gist: instantly share code, notes, and snippets. Slot machine with 3 rolling wheels, every wheel can show one of the two symbols avaiable (Symbols are leds). Three push button can controls the 3 wheels and the starts of the game. In the case of win the leds show an a specific combination.
A Tkinter GUI Slot Machine Project
This project is still in its early stages, but it’s currently playable.
This version of the game is lacking several features that I have planned for it, and there may be memory issues, if played for a long time, I’m not sure about that yet though.
As always, my code leaves a lot to be desired, especially at this stage of development where I don’t care that much about efficiency, just weather it works or not, this is really bad practice I know, so keep that in mind please.
Features So Far
- Only uses standard library (so far)
- It’s called Freespin Frenzy because once you get the freespins feature up you can potentially win a (virtual) fortune with unlimited re-triggers.
- Auto saves high-score, and reloads it the next time you play.
- Hold buttons with random chance of hold after win.
- All wins are tripled during freespins.
- Select stakes, $1-$5 per spin.
Look familiar? That’s because I used the base code from my Poker Machine game for now. I will most likely redo the buttons, change the look and feel, and I’m also considering getting rid of the playing cards and using my own custom symbols, not sure about that yet though.
I may try for five reels at some stage, but I fear that it will get too complex for my little brain. I have left room for a reel either side of the three reels for now, I will either fill those spaces in with something, or do five reels if I’m capable.
Planned Features
This version is already a dial-back. I had spent a few days adding a freespin accumulator feature, where during the course of a normal game any freespin card that came up on your pay-line would add one to the accumulator, when you reached 10 or more you got 10 freespins on normal payout (not tripled).
Dial Back
I had lots of problems with it, but the meter window that I tacked onto the bottom of the GUI would not update nicely and a few other problems that really peeved me off, so in the end I scrapped it and returned to this version.
Code Of Note
The only bit of code worth noting so far, I think, is due to Pylint and pycodestyle, they both pointed out a particularly bad bit of code, (and there’s plenty of it to choose from) and suggested a better way, ah, that’s nice innit. So I had a go, and it worked out great, much to my surprise.
Boolean monstrosity
I was using this code to detect wins. This monstrosity checks for all possibilities of a jacks win, the ‘W’ is the wild card.
Pylint suggested this:
So I took a look at it and played about with “in”, if out_come in “JJJ” or “WJJ” etc.
But this didn’t work. I then realised that I had to put these win combos into a list, I probably should have done that from the start of course!
Wow, that’s much better code, very clear, clean and concise.
Payout Percentage?
At this stage I am just guessing what the prizes should be. At first I wanted a 5001 jackpot, but because of potential hold after win and the chance of getting the triple jackpot during freespins I had to cut that to 2001 and make sure that if the player achieved the jackpot during freespins, which is three wildcards, it would end the feature.
I could of said hang it, it doesn’t have to be realistic, and it probably still isn’t a realistic payout, but I have to weigh up fun with realism and play-ability.
If you played the game and didn’t win anything or get the feature up for ages, that wouldn’t be much fun in my book, striking the right balance will be difficult because if winning is too easy, then what is the point?
Memory Fix
As for the out of memory problem I mentioned earlier, I think that has to do with me loading each card in as and when required, which is a bad way of doing things, and I will be looking at fixing that next.
Possible future features
- I really want the freespin accumulator, and may try again but make it simpler.
- I have an idea for a “spoiler card” on reel 3 that resets the freespin accumulator, otherwise it is far too easy to get the feature up, I may also increase the meter from 10 to 25.
- Bonus pot. I have this written down in my plan but I have not tried to implement it yet. It would probably start off at x100 stake and then add 5% of each stake played to the pot, although these figures will probably need to be adjusted to reality. Three bonus pot cards (which I have yet to create) together on the reels would win the pot. The pot prize would be paid for by the 5% of stakes and the fact that the bonus pot cards would stop a lot of wins coming in by getting in the way. These ideas are all open to change of course.
- Matching suits. If all three cards are diamonds for example you get your stake back, hmm, maybe adds a little to the game and helps break up long boring runs if no wins are about. This would be at minimal cost to the payout percentage. It would still be tripled during freespins. I’m fairly certain I will be adding this feature.
- I am now considering making the jackpot only x100 stake, but also adding the freespin feature to it, double joy? This might only pay out normally, not triple wins though.
- Freespin accumulator feature may payout double wins.
- I would dearly love to have a spinanimation just like a real slot machine, but there is no way that I could code it, and I doubt anyone will come forward with a solution, hint, hint.
- Music and sound effects.
- My usual drop-down menu.
- I have more ideas, but most are probably too far off my coding ability.
Feel free to mess about with the code, or just enjoy playing the game if you want.
There are comments in the code to cheat, for testing purposes.
Download The Code
Get the V0.64 source code and associated files in a zip from MediaFire, 150k.
Get the V0.64 source code and associated files AND Windows executable in a zip from MediaFire, just 8mb.
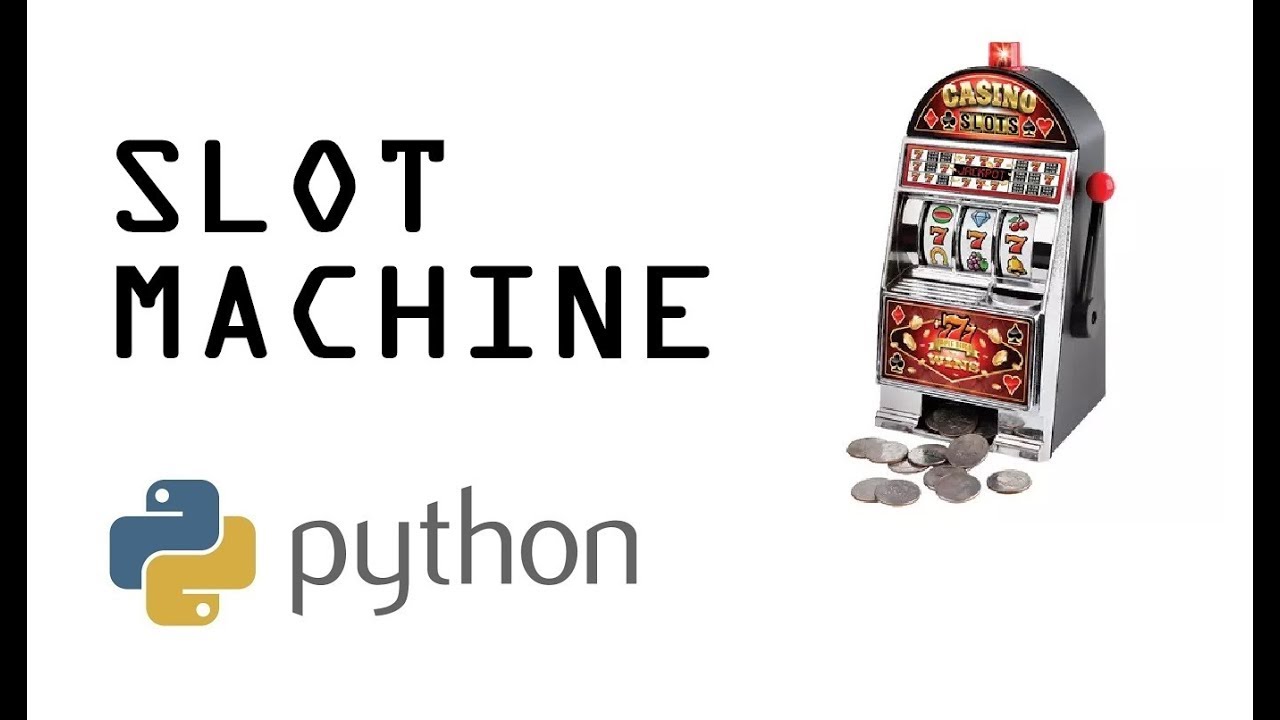
NOTE: V0.79 is now available from GitHub or from GUI Slots V0.79 Update page.
Tiny Files
Note the tiny file sizes, this is, I assume, because I haven’t, as yet, used a third party module, just the standard library.
Watch out for future updates.
- I haven’t tested this on Linux yet. It should work though.
Steve.
Python Slot Machine Gui Pastebin
Using Python V3.7.2 32bit, on Windows 7 64bit
and Python V3.67 on Linux Mint 19.1 Cinnamon 64bit
Slot Machine For Sale
Next Post: Python Code Snippets Vol.55
Previous post: Infi-Systray Separator Bar
PyQt5 supports several input dialogs, to use them import QInputDialog.
An overview of PyQt5 input dialogs:
Related course:
Get integer
Get an integer with QInputDialog.getInt():
Parameters in order: self, window title, label (before input box), default value, minimum, maximum and step size.
Get double
Get a double with QInputDialog.getDouble():
The last parameter (10) is the number of decimals behind the comma.
Get item/choice
Get an item from a dropdown box:
Get a string
Get a string using QInputDialog.getText()
Example all PyQt5 input dialogs
Complete example below:
If you are new to programming Python PyQt, I highly recommend this book.